Tools API
This article describes all available options for Tools creation. See «Creating a Block Tool» or «Creating an Inline Tool» guide series for usage examples and more detailed explanations.
Each Tool can be implemented by a class. It's constructor accepts three useful arguments:
data
|
Previously saved data. Can be used to update UI state on article editing. |
api
|
Editor.js Core API object |
config
|
User configuration object that passed through the initial Editor configuration. |
readOnly
|
The flag determines whether the read-only mode enabled or not. You should disable data modifications ability and change the UI as well in this mode. |
block
|
BlockAPI object with methods to work with Block instance |
render
|
Required | Creates UI of a Block |
save
|
Required | Extracts Block data from the UI |
validate
|
Optional |
Validates Block data after saving. If returns false , Block will be skipped on Editor saving.
|
renderSettings
|
Optional | Returns configuration for the tunes that will be displayed in Block Tunes menu |
destroy
|
Optional | Contain logic for clear Tools stuff: cache, variables, events. Called when Editor instance is destroying. |
onPaste
|
Optional |
Handle content pasted by ways that described by pasteConfig static getter.
|
merge
|
Optional | Method specifies how to merge two similar Blocks |
pasteConfig
|
Allows your Tool to substitute pasted HTML tags, files or URLs. |
sanitize
|
Automatic sanitize configuration. Allows to clean unwanted HTML tags or attributes from files with Inline Toolbar. |
toolbox
|
Required if Tools should be added to the Toolbox. Describe an icon and title here.
|
shortcut
|
Shortcut that fires render method and inserts new Block |
conversionConfig
|
Config allows Tool to specify how it can be converted into/from another Tool. |
enableLineBreaks
|
With this option, Editor.js won't handle Enter keydowns. Can be helpful for Tools like Code where line breaks should be handled by default behavior. |
isReadOnlySupported
|
This flag tells core that current tool supports the read-only mode. |
Define these public methods to handle any state of Block's lifecycle
rendered
|
Called after Block contents is added to the page |
updated
|
Called each time Block contents is updated |
removed
|
Called after Block contents is removed from the page but before Block instance deleted |
moved(event: MoveEvent)
|
Called after Block is moved by move tunes (or through API). MoveEvent extends CustomEvent interface, its details field contains the object with fromIndex and toIndex properties.
|
Create a Tool UI here. Should return single HTML Element that contains all your interface.
This method has no arguments.
Element
|
Tool's UI wrapper |
Don't forget to fill the UI with previously saved data passed to class constructor.
Only for Block Tools.
Inversely to the render
method, it should extract actual data from the UI and return a Promise returned data of the Block.
Element
|
Element created by render method.
|
Promise.<Object> or Object
|
Block data to save. |
Optional method.
Validates saved data to skip invalid (for example, empty) Blocks.
Object
|
Data returned by save method.
|
Boolean
|
Validation result. Return false to skip Block or true if data is correct.
|
Method allows to define block tunes. Can either return Tunes Menu configuration or single HTML element with block tunes UI elements.
This method has no arguments
TunesMenuConfig
|
Configuration of tunes appearance inside Block Tunes menu |
Element
|
Single element that contains a Block Settings UI elements. |
Method will be fired when Editor's instance is destroying with destroy
API method. Clear your Tool's stuff here: remove event listeners, clear nodes cache and null variables and properties.
This method has no arguments
This method should not return anything.
After you set a pasteConfig
, for each of paste substitutions (tags, files, and patterns) you need just one method — onPaste
. It accepts CustomEvent
object as argument which can have three types: tag
, file
, and pattern
. Each event provides info about pasted content in detail
property.
-
Tag
event contains pasted HTML element indetail.data
property. -
File
event contains pasted file object indetail.file
property.
-
Pattern
event contains pasted string indetail.data
property and pattern name indetail.key
.
CustomEvent
|
Custom paste event with detail property.
|
This method should not return anything.
Method that specifies how to merge two similar Blocks, for example on Backspace
keypress. It accepts data object in same format as the Render
and it should provide behaviour how to combine new data with the currently stored value.
Object
|
Block data in same format that returned by save method
|
This method should not return anything.
Static getter pasteConfig
shoud return object with paste substitutions configuration:
-
tags
— array of tags to substitute -
files
— object with mimeTypes and extensions arrays -
patterns
— object where key is your pattern name and RegExp pattern as value
{tags: string[], files: { mimeTypes: string[], extensions: string[] }, patterns: { [string]: RegEx }}
|
Paste configuration. |
For example for Image Tool we need to substitute img tags, handle files with image/* MIME-type and handle URLs to images:
Then you need to specify onPaste handler to implement logic how to make your Tool from pasted content. See more information about paste substitution here.
Object that defines rules for automatic Sanitizing.
Object
|
Sanitizer configuration rules for each of saved field. |
Defines icon
and title
of the Tool's button inside the Toolbox. This getter is required for Block Tools that should be rendered in the Toolbox.
{icon: string, title: string}
|
Icon and Title for the Toolbox |
{icon: string, title: string, data: any}[]
|
In multiple entries case returns array of each entry configuration. Note the data field — it should contain data overrides that will be passed to the Block constructor. The data object should fit the structure of the Block’s output data or be a part of it.
|
To render several variants for one Tool in the Toolbox, toolbox
getter should return an array:
Such toolbox configuration will make the Tool appear in the Toolbox multiple times which will look as follows
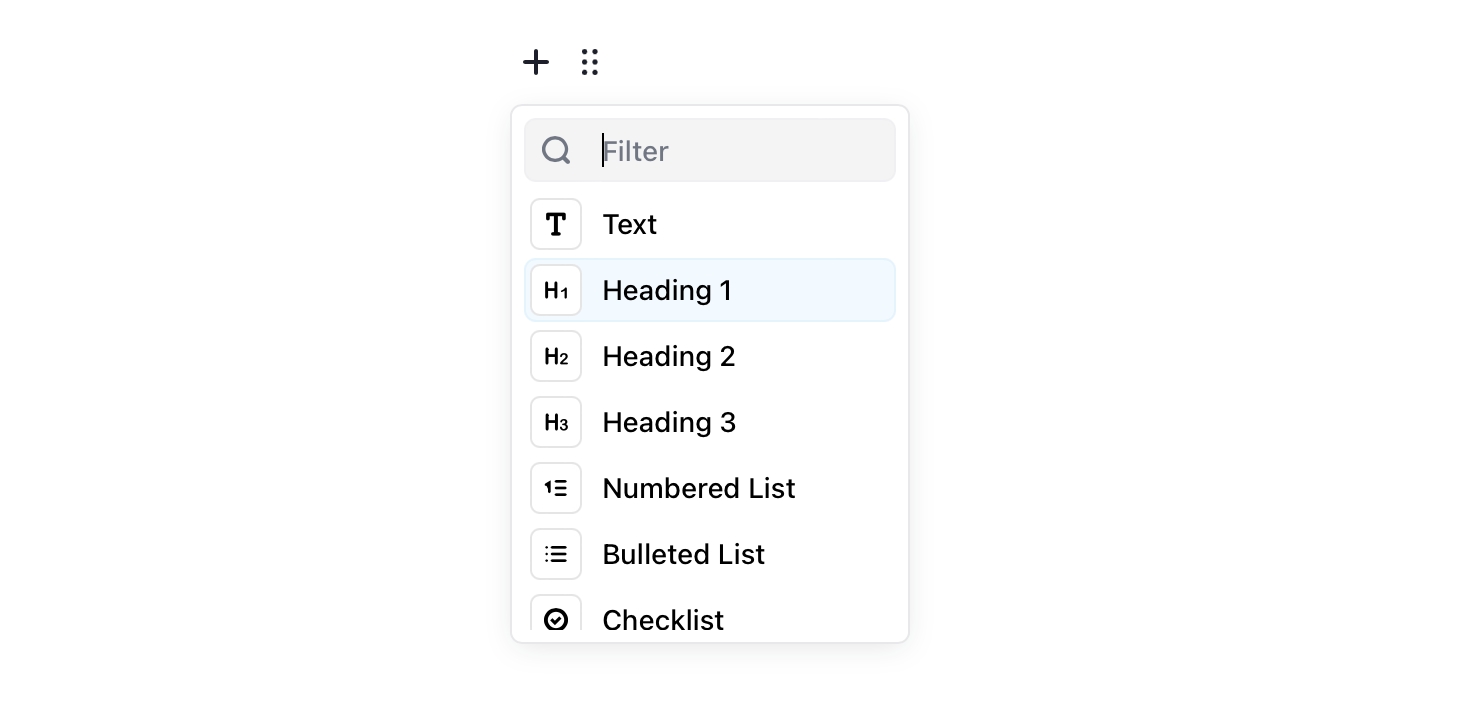
Shortcut to apply Tool's render and inserting behaviour
String
|
Shortcut for Tool inserting. Format described here. |
Editor.js has a Conversion Toolbar that allows user to convert one Block to another.
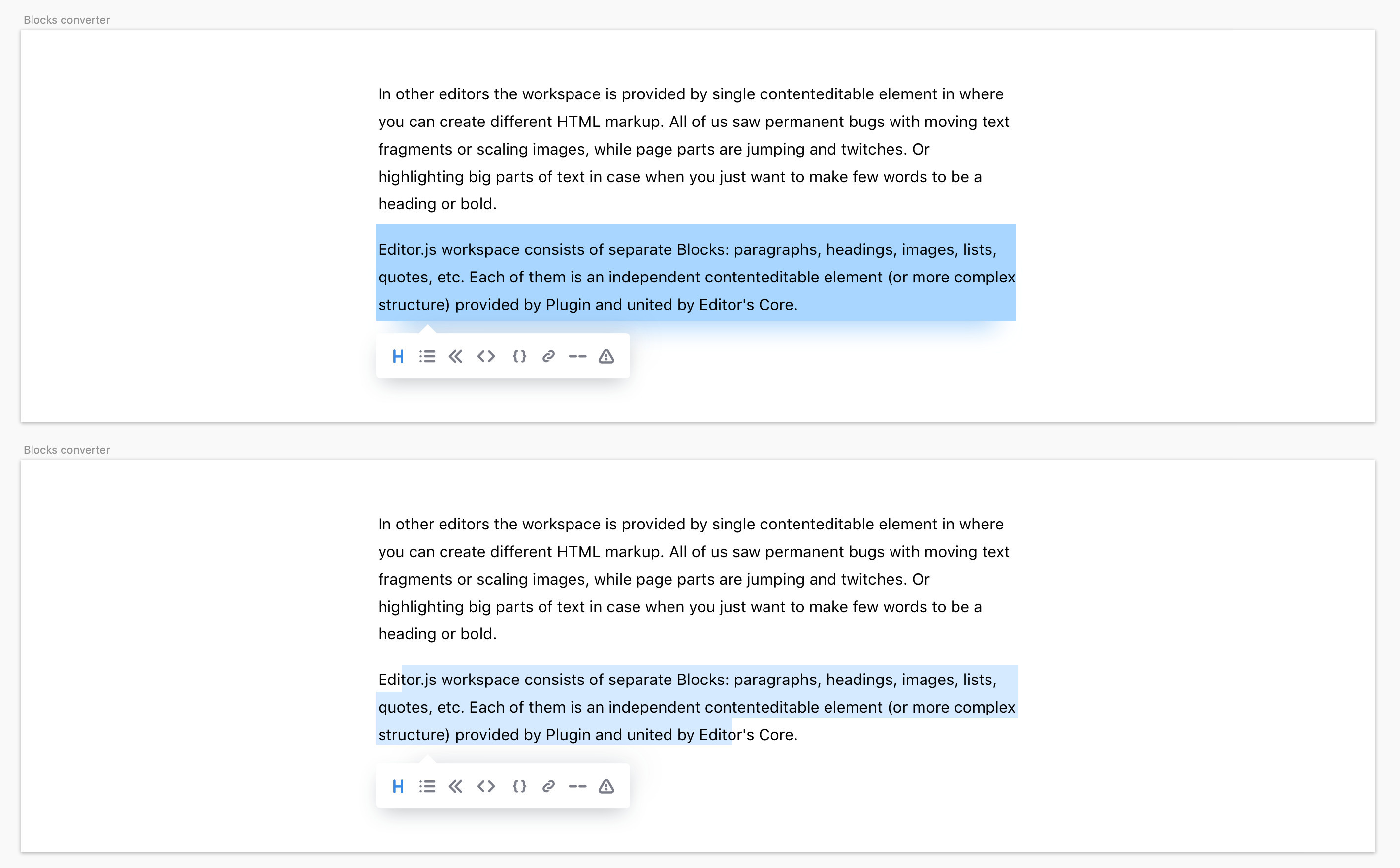
-
You can add ability to your Tool to be converted. Specify «export» property of
conversionConfig
-
You can add ability to convert other Tools to your Tool. Specify «import» property of
conversionConfig
The «export» field specifies how to represent your Tool's data as a string to pass it to other tool.
It can be a String
or a Function
String
means a key of your Tool data object that should be used as string to export.
Function
is a method that accepts your Tool data and compose a string to export from it. See example below:
he «import» rule specifies how to create your Tool's data object from the string created by original block.
It can be a String
or a Function
String
means the key in tool data that will be filled by an exported string.
For example,
means that import: 'text'
constructor
of your block will accept a
object with data
property filled with string composed by original block.
text
Function
allows you to specify own logic, how a string should be converted to your tool data. For example:
With this option, Editor.js won't handle Enter keydowns. Can be helpful for Tools like Code where line breaks should be handled by default behavior.
Boolean
|
If true , Editor won't handle Enter keydowns for this tool
|
Use this flag to tell the editor that your tool can be rendered in the read-only mode
Boolean
|
If true , editor.js can be rendered with the read-only mode with your tool
|